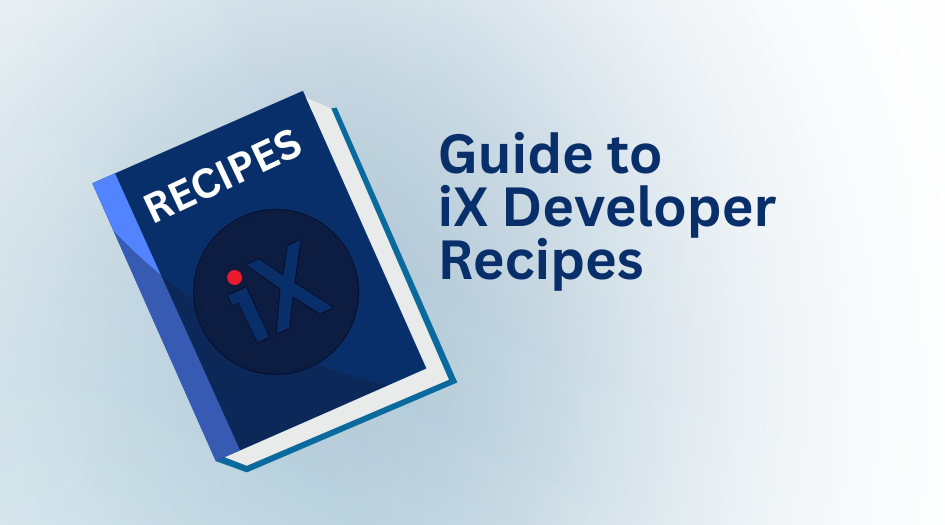
Contents
ToggleWhat is a Recipe in iX Developer?
A recipe in iX Developer is a predefined set of parameter values for different operational scenarios. For instance, in a food-processing line, you might have different recipes for “Chocolate Cake,” “Vanilla Cake,” and “Banana Cake,” each specifying temperature, mixing speed, and timer settings.
- Recipe: A named collection of parameters (tags).
- Parameter: Each data item (tag) within the recipe (e.g., setpoint, mixing speed, temperature).
- Recipe Manager: A built-in control in iX Developer to display, edit, load, and save recipes at runtime.
Legend:
- Recipe: A predefined set of parameter values (tags) for a particular process or product.
- Recipe Group: A way to organize multiple recipes.
- Recipe Row: A single “instance” or variant of a recipe (e.g., “Chocolate Cake”).
- Parameter (Tag): Individual data item within a recipe (e.g., Temperature, Speed).
- Recipe Manager: A built-in control to visualize, load, save, and edit recipes at runtime.
- C# Scripting: Allows custom logic for advanced recipe loading/saving scenarios.
Download an iX Developer Recipe Example Project
Complete the form and we’ll send you a download link by email.
How to Create a Recipe Structure
- Open or create a project in iX Developer.
- In the Insert toolbar click the Recipe button to insert a recipe into your project.
- Rick click the new recipe in the file explorer and rename your recipe something descriptive, e.g., CakeRecipe.
- In the Recipe Editor window that appears, you can define columns or fields for each parameter in your recipe (e.g., Temperature, MixingSpeed, BakingTime).
Tip
- If you have multiple recipe variants (e.g., “Chocolate Cake,” “Vanilla Cake,” etc.), you can add rows in your recipe table, each row representing a different variant.
- If you have multiple sets of parameters that logically belong together (e.g., “Oven Settings,” “Mixing Settings”), you may want to create multiple recipes or use multiple columns in a single recipe.
Defining Recipe Parameters (Tags)
Each parameter within the recipe links to one or more tags. Typically, these tags correspond to machine setpoints or process variables.
- Still in the Recipe Editor, locate the column for your parameter, e.g., Temperature.
- Under the Tag column (or parameter properties), browse to select the corresponding tag (e.g., OvenTemperature from your project’s tag list).
- Repeat for other columns, such as MixingSpeed → MixerSpeedTag, BakingTime → BakingTimeTag, etc.
- Save your recipe configuration.
Result
- Each row in your recipe table (e.g., “Chocolate Cake”) has a set of default values for these tags.
- During runtime, loading a row automatically writes those values into the linked tags, effectively setting your machine parameters.
Building a Recipe Screen
To allow operators to interact with recipes, create or open a screen in your project:
- In the Project Explorer, right-click Screens → Add Screen → Name it RecipeScreen.
- Open RecipeScreen by double-clicking it.
- From the Toolbox, drag controls that will be used to display and modify recipe data:
- Text Controls to display parameter names.
- Numeric Entry or Text Entry controls (if you want to allow editing of parameters).
- Buttons for loading, saving, or navigating.
Loading/Saving Recipes at Runtime (Programmatic Approach)
If you prefer more control or a custom UI, you can load and save recipes programmatically with C#.
Loading a Recipe
Suppose you have a button named btnLoadChocolateRecipe. In the code-behind, you might do:
using System;
using Neo.ApplicationFramework.Tools.Recipe;
using Neo.ApplicationFramework.Interfaces;
namespace Neo.ApplicationFramework.Generated
{
public partial class RecipeScreen
{
void btnLoadChocolateRecipe_Click(object sender, EventArgs e)
{
// "CakeRecipe" is the name of the recipe in iX Developer
// "Chocolate Cake" is the row name within that recipe
var recipeSystem = RecipeSystem.Instance;
recipeSystem.LoadRecipe("CakeRecipe", "Chocolate Cake");
}
}
}
When the button is clicked, it loads the row named “Chocolate Cake” from the recipe named “CakeRecipe,” writing all parameter values to the linked tags.
Alternatively you can select Actions for the button, choose Load Recipe and select the recipe you with to load.
Saving a Recipe
If you have adjusted parameters at runtime and want to update the recipe row, use:
void btnSaveChocolateRecipe_Click(object sender, EventArgs e)
{
var recipeSystem = RecipeSystem.Instance;
recipeSystem.SaveRecipe("CakeRecipe", "Chocolate Cake");
}
Example Scenarios / Common Use Cases
Food & Beverage
- Different recipes for each product variant (e.g., different flavor profiles).
- Loading a recipe sets the temperature, mixing speed, and time for that product run.
- Batch Manufacturing
- Quickly switch parameters between product A and product B (e.g., fluid levels, conveyor speeds).
- Packaging Industry
- Different bag sizes require different machine settings (e.g., label position, bag length).
- A recipe can store settings for each bag size.
- Metal Cutting / Machining
- Save feed rate, spindle speed, coolant rate for each type of material (e.g., aluminum, steel, plastic).
- Printing Press
- Store ink densities, roller speeds, tension settings for various print jobs.
Summary
Recipes in iX Developer simplify the process of loading and saving multiple tag values as a group, enabling quick and repeatable machine setup changes. By creating a recipe table linked to your tags, you can provide operators with a straightforward interface—either through the built-in Recipe Manager control or via custom-coded load/save buttons.
Next Steps
- Experiment with different recipe structures and parameter types (numeric, boolean, text).
- Combine recipes with security settings so only authorized users can modify or load certain recipes.
- Add code-based or triggered events to automatically load recipes when certain conditions are met (e.g., new product order).
With these approaches, you can effectively streamline machine setup, reduce errors, and speed up changeovers using iX Developer’s recipe functionality.